
P5.js Sketches
P5.JS SKETCHES
Click on the headings to run the sketches. Enjoy!

TRANSPARENCY
function setup() {
createCanvas(1440, 800);
background (50);
}
function draw() {
stroke('red');
fill(255, 240, 0, 40);
circle(mouseX, mouseY, 150);
}
function mousePressed() {
background (50);
}
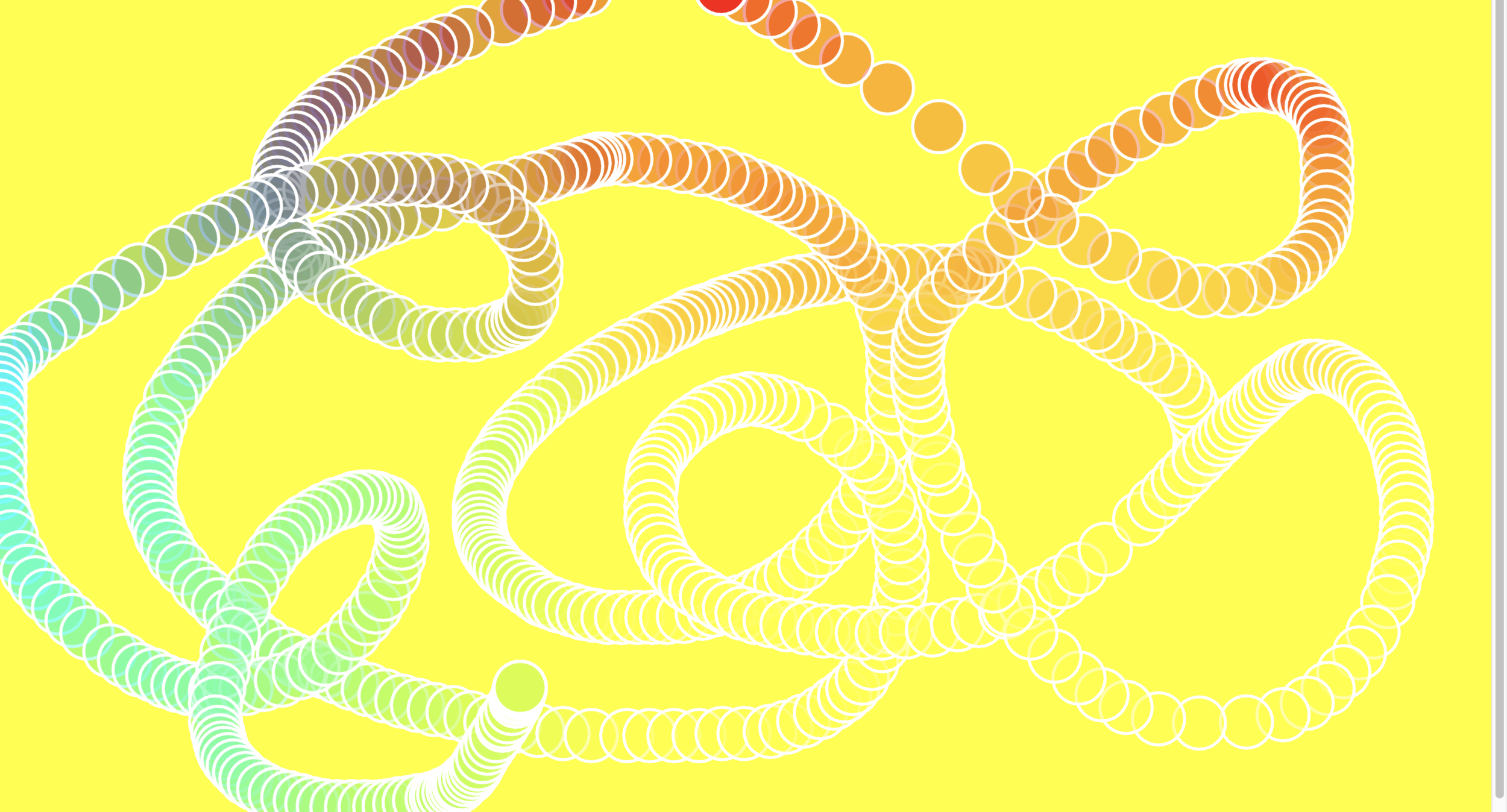
DISCO DANCE 1.0
var r = 0;
var g = 0;
var b = 255;
function setup() {
createCanvas(1440, 800);
background(r, g, b);
}
function draw() {
r = map(mouseX, 0, 600, 0, 255);
g = map(mouseY, 0, 400, 0, 255);
b = map(mouseX, 0, 600, 255, 0);
fill(r, g, b, 100);
stroke('white');
strokeWeight(3);
ellipse(mouseX, mouseY, 50, 50);
}
function mousePressed() {
background(r, g, b);
}
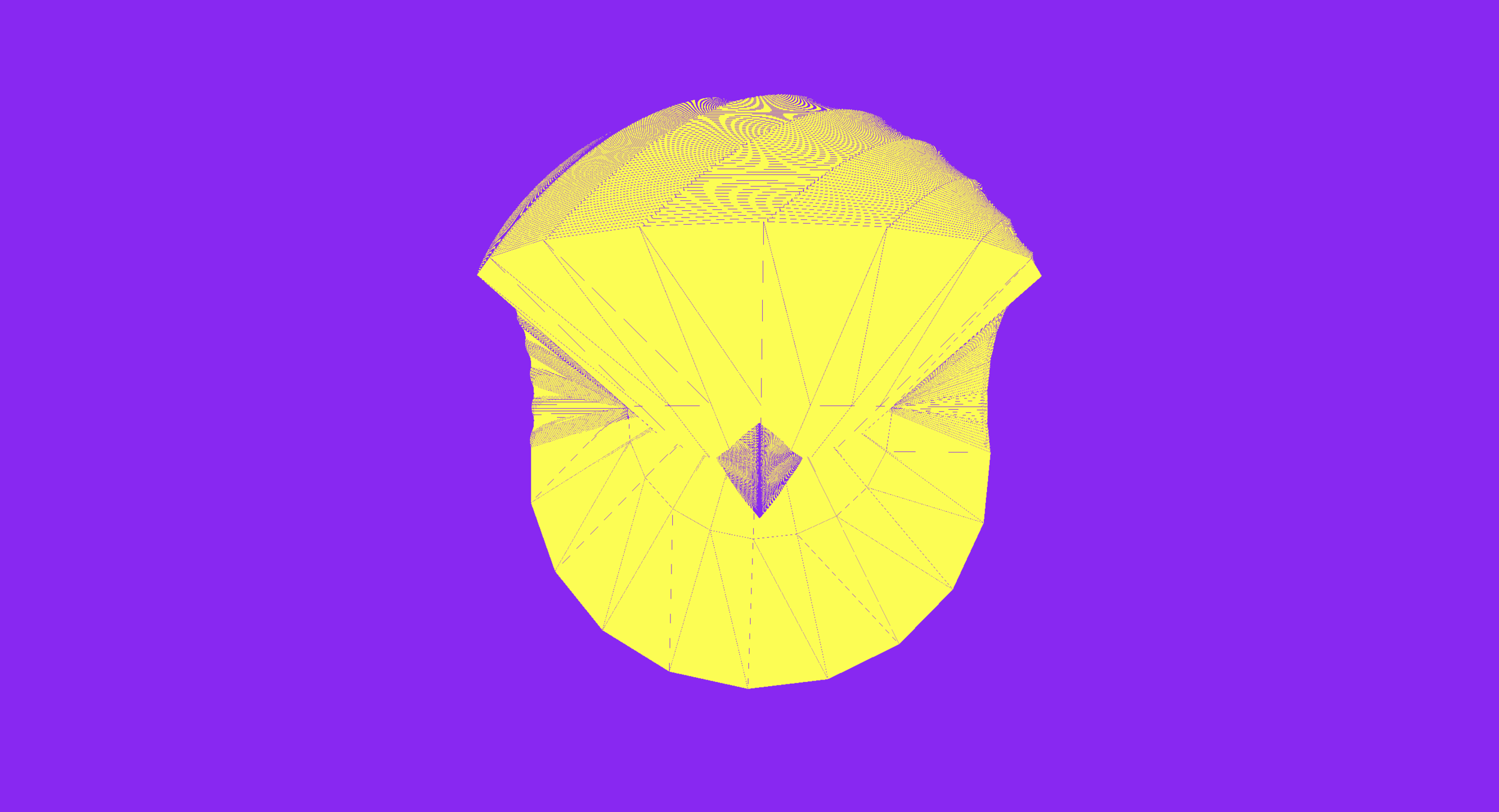
TORUS
// draw a spinning box
// with width, height and depth of 50
function setup() {
createCanvas(1440, 800, WEBGL);
background(150, 0, 250);
}
function draw() {
stroke(150, 0, 250);
strokeWeight (0.2);
fill(250, 280, 0);
rotateX(frameCount * 0.01);
rotateY(frameCount * 0.01);
cone(250, 220, 20, 2);
}

QUAD ARROW
function setup() {
createCanvas(1440, 800);
}
function draw() {
fill(255, 0, 0);
quad(mouseX, mouseY, mouseX + 150, mouseY + 15, mouseX + 10, mouseY + 10, mouseX + 15, mouseY + 15, );
}
function mousePressed(){
clear();
}

TRACES
//circle
var circle1 = {
x: 720,
y: 800,
d: 60
}
//rectangle 2
var rect2 = {
x: 600,
y: 0,
l: 80,
b: 50
}
//square
var square1 = {
x: 830,
y: 0,
s: 65
}
var r = 250
var g = 180
var b = 10
var a = 10
function setup() {
createCanvas(1440, 800);
}
function draw() {
background(r, g, b, a);
//circle
fill('green');
noStroke();
circle(circle1.x, circle1.y, circle1.d);
circle1.y = circle1.y - 1
circle1.d = circle1.d + 0.1
//rectangle 2
fill('red');
noStroke();
rectMode(CENTER);
rect(rect2.x, rect2.y, rect2.l, rect2.b);
rect2.y = rect2.y + 1
//square
fill('blue');
noStroke();
square(square1.x, square1.y, square1.s);
square1.y = square1.y + 1
}
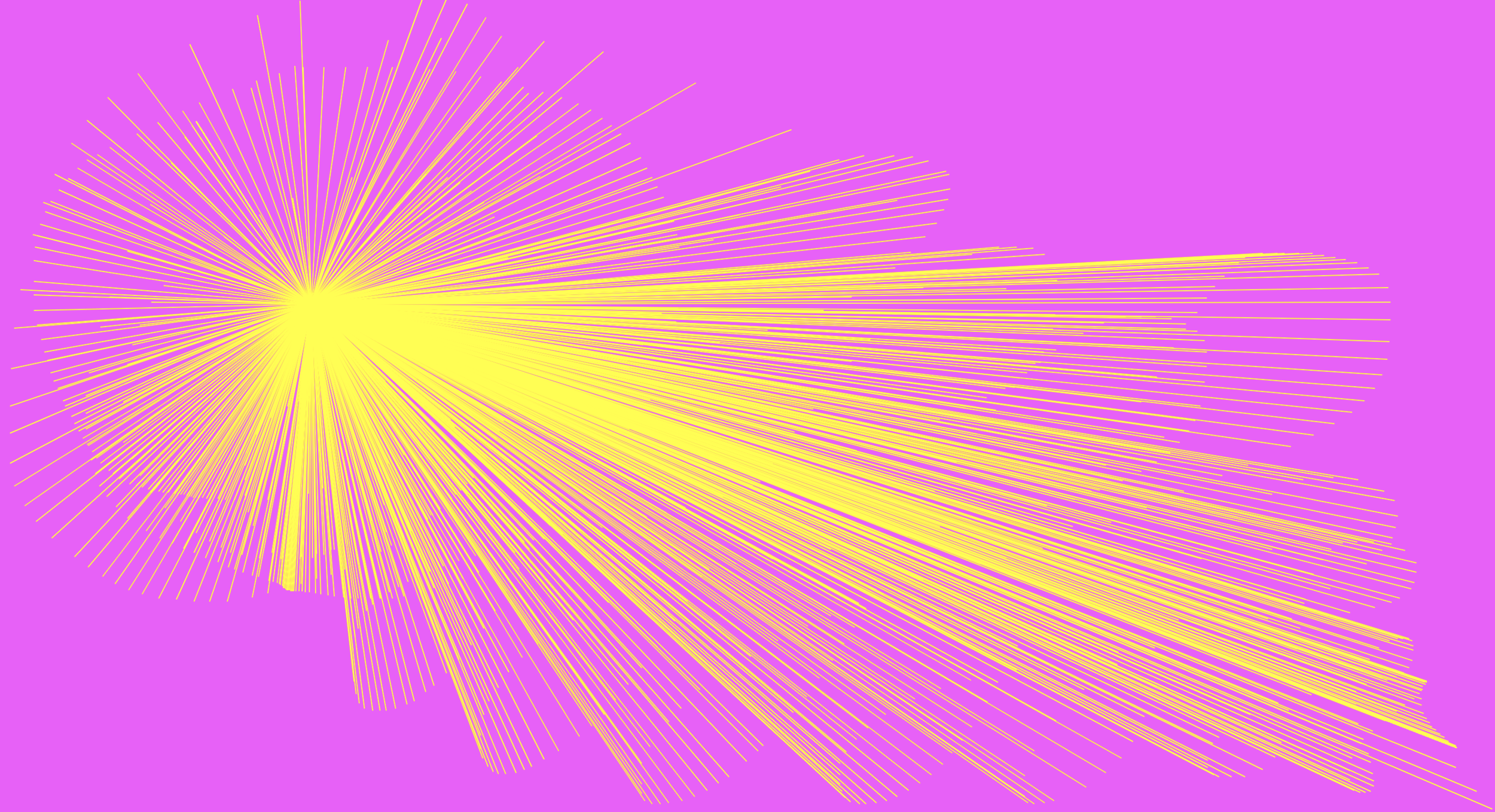
LINE ARROW
function setup() {
createCanvas(1440, 800);
background (250, 80, 255);
}
function draw() {
stroke('yellow');
line(mouseX, mouseY, 300, 300);
}
function mousePressed(){
background (250, 80, 255);
}
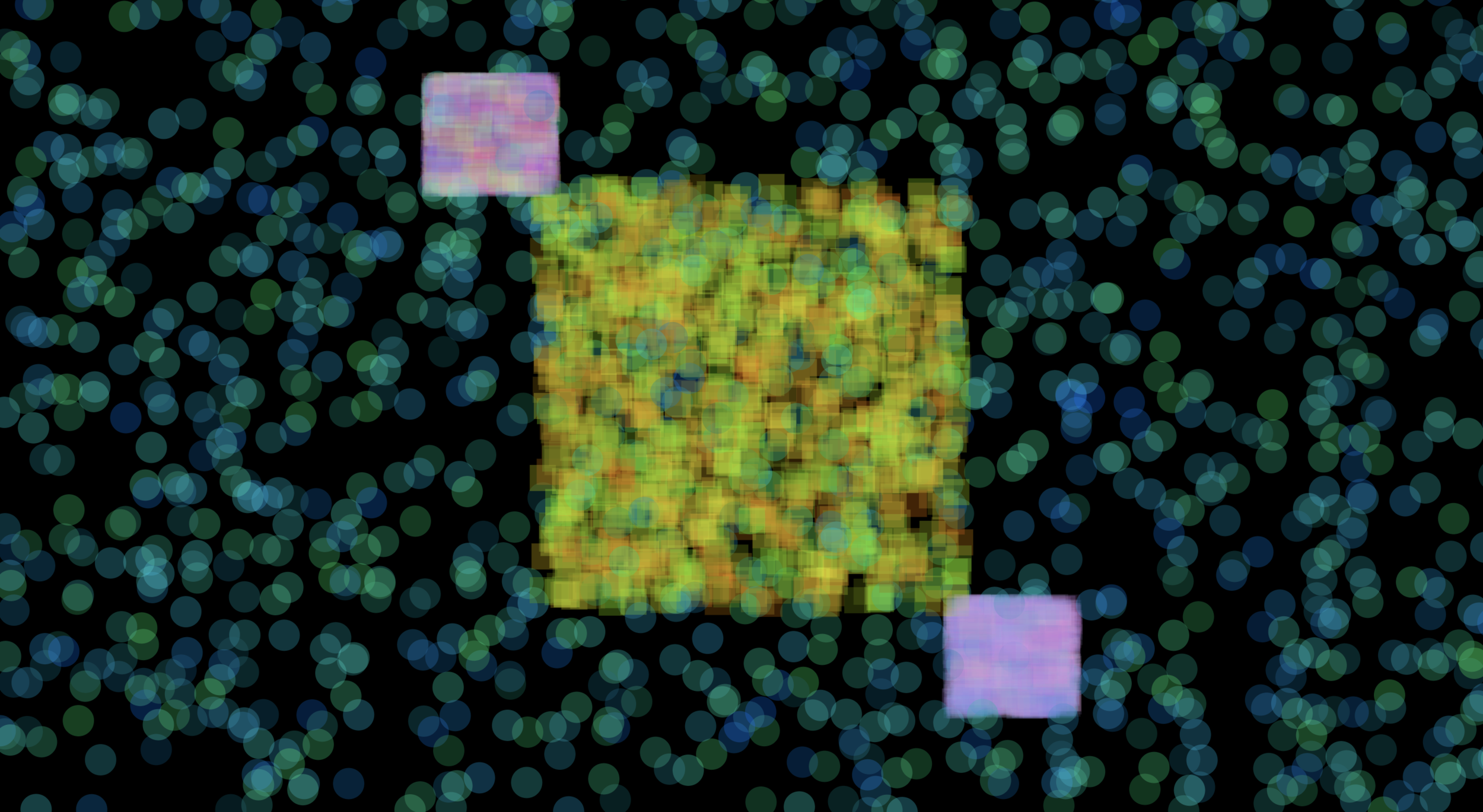
RANDOM
var point1 = {
x: 720,
y: 400
}
var col = {
r: 250,
g: 10,
b: 40
}
function setup() {
createCanvas(1440, 800);
background(0);
}
function draw() {
col.r = 0;
col.g = random(100, 255);
col.b = random(100, 255);
point1.x = random(0, width);
point1.y = random(0, height);
fill(col.r, col.g, col.b, 70);
noStroke();
ellipse(point1.x, point1.y, 30, 30);
col.r = random(100, 255);
col.g = random(100, 255);
col.b = 0;
point1.x = random(520, 920);
point1.y = random(200, 600);
fill(col.r, col.g, col.b, 70);
noStroke();
rectMode(CENTER);
square(point1.x, point1.y, 25);
col.r = random(100, 255);
col.g = random(40, 255);
col.b = random(100, 255);;
point1.x = random(420, 520);
point1.y = random(100, 200);
fill(col.r, col.g, col.b, 40);
noStroke();
rectMode(CENTER);
rect(point1.x, point1.y, 35, 20);
col.r = random(100, 255);
col.g = random(100, 190);
col.b = random(200, 240);
point1.x = random(920, 1020);
point1.y = random(600, 700);
fill(col.r, col.g, col.b, 30);
noStroke();
rectMode(CENTER);
rect(point1.x, point1.y, 35, 20);
// col.g = map (mouseX, 0, 600, 0, 255);
}
function mousePressed() {
background(col.r, col.g, col.b);
col.r = map(mouseX, 0, 600, 0, 255);
col.g = map(mouseX, mouseY, 400, 0, 255);
col.b = map(mouseX, 0, 600, 255, 0);
}
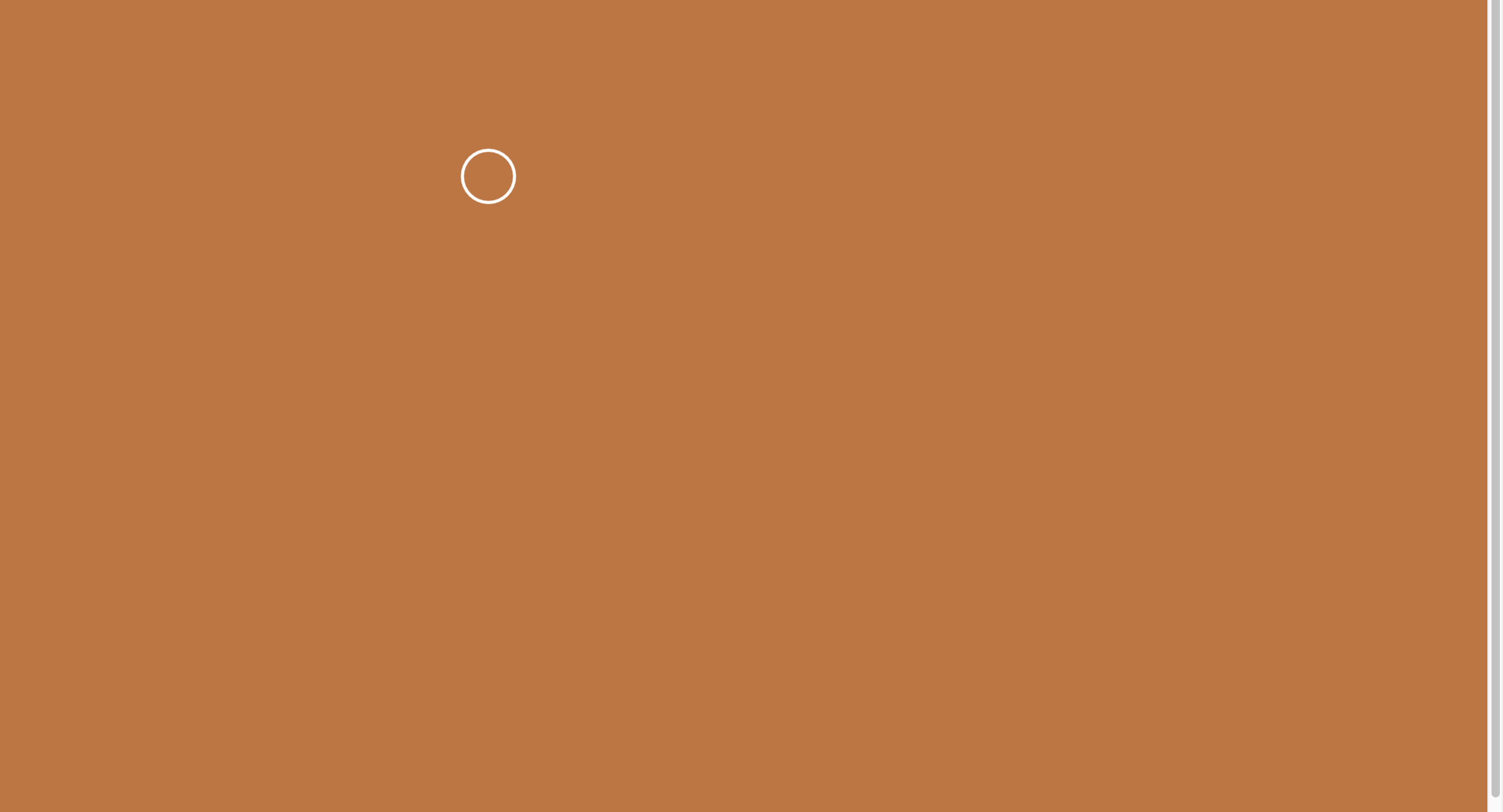
DISCO DANCE 2.0
var r = 0;
var g = 0;
var b = 255;
var circle1 = {
x: 0,
y: 300,
d: 20
}
function setup() {
createCanvas(1440, 800);
}
function draw() {
background(r, g, b);
r = map(mouseX, 0, 600, 0, 255);
g = map(mouseY, 0, 400, 0, 255);
b = map(mouseX, 0, 600, 255, 0);
fill(r, g, b, 50);
stroke('white');
strokeWeight(3);
ellipse(circle1.x, circle1.y, circle1.d, circle1.d);
circle1.x = mouseX
circle1.y = mouseY
if (mouseX > 200) {
circle1.d = 30
}
if (mouseX < 200) {
circle1.d = 20
}
if (mouseX > 300) {
circle1.d = 40
}
if (mouseX > 400) {
circle1.d = 50
}
if (mouseX > 500) {
circle1.d = 60
}
}
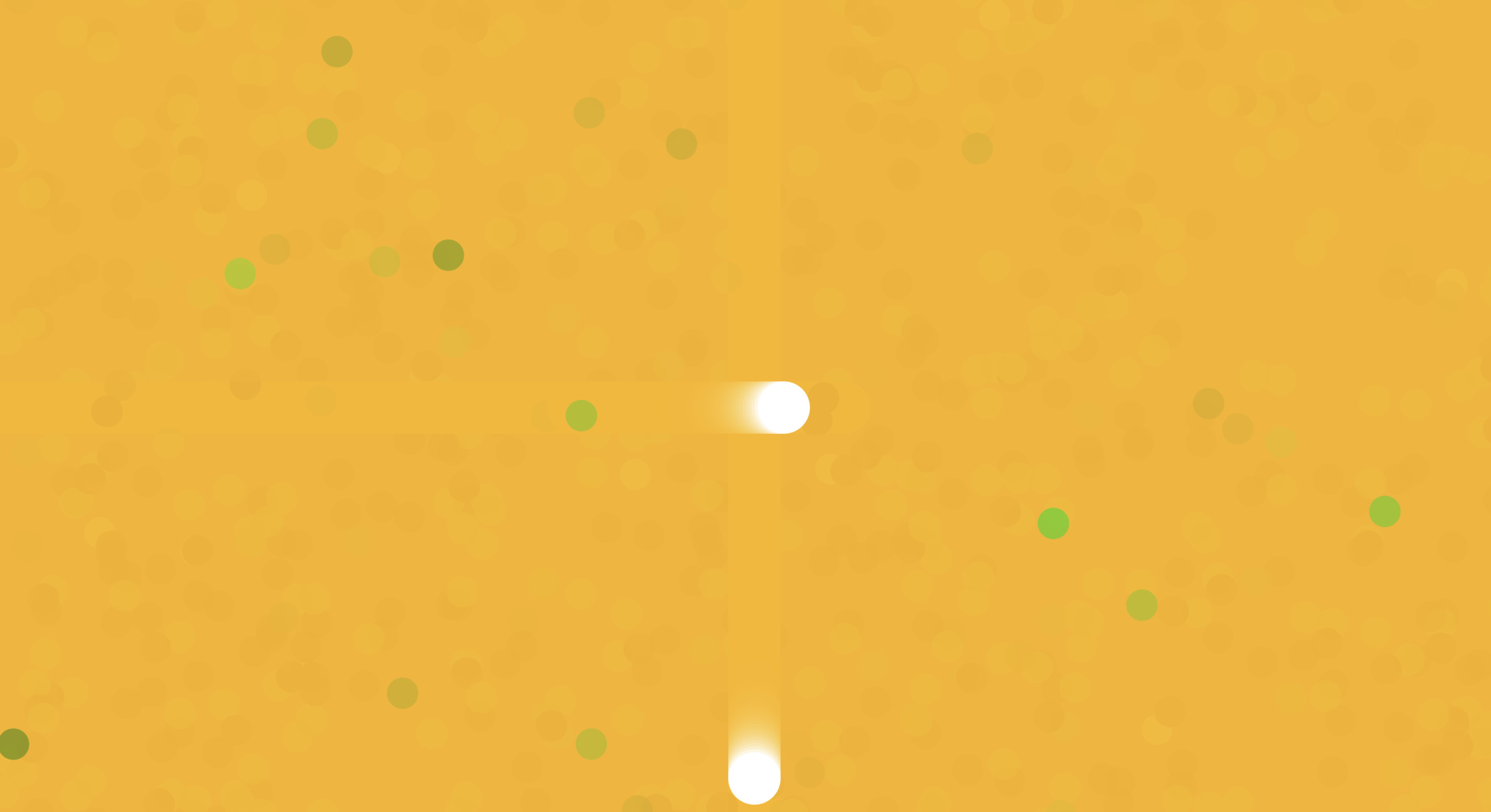
BOUNCING BALL
var x = 0
var y = 0
var speed = 5
var dot = {
x: 200,
y: 300
}
var a = 250;
var d = 180;
var h = 0;
function setup() {
createCanvas(1440, 800);
//background(250, 180, 0);
}
function draw() {
background(250, 180, 0, 30);
//ellipse 1
fill(255);
noStroke();
strokeWeight(3);
ellipse(x, 400, 50, 50);
x = x + speed
if (x > width || x < 0) {
speed = speed * -1;
}
//ellipse2
fill(255);
noStroke();
strokeWeight(3);
ellipse(720, y, 50, 50);
y = y + speed
if (y > height) {
speed = -3;
}
//random circles
r = 0;
g = random(100, 250);
b = 0;
dot.x = random(0, 1440);
dot.y = random(0, 800);
fill(r, g, b, 120);
noStroke();
circle(dot.x, dot.y, 30);
}

COLOR COLLISION
//rectangle 1
var rect1 = {
x: 0,
y: 300,
l: 15,
}
//rectangle 2
var rect2 = {
x: 520,
y: 0,
l: 25,
}
//rectangle 3
var rect3 = {
x: 1440,
y: 100,
l: 40,
}
//rectangle 4
var rect4 = {
x: 900,
y: 800,
l: 70,
}
function setup() {
createCanvas(1440, 800);
background('white');
}
function draw() {
fill('white');
stroke('black');
rectMode(CENTER);
rect(mouseX, mouseY, 40, 40);
// function draw() {
strokeWeight(0.5);
// background('white');
//rectangle 1
fill('pink');
stroke('black');
rectMode(CENTER);
rect(rect1.x, rect1.y, rect1.l, rect1.l);
rect1.x = rect1.x + 2
rect1.l = rect1.l + 0.05
//rectangle 2
fill('yellow');
stroke('black');
rectMode(CENTER);
rect(rect2.x, rect2.y, rect2.l, rect2.l);
rect2.y = rect2.y + 2
rect2.l = rect2.l + 0.05
//rectangle 3
fill('green');
stroke('black');
rectMode(CENTER);
rect(rect3.x, rect3.y, rect3.l, rect3.l);
rect3.x = rect3.x - 2
rect3.l = rect3.l - 0.05
//rectangle 4
fill('lavender');
stroke('black');
rectMode(CENTER);
rect(rect4.x, rect4.y, rect4.l, rect4.l);
rect4.y = rect4.y - 2
rect4.l = rect4.l - 0.1
}
function mousePressed() {
background('white');
}